Christmas Day is every bit as magical for the parents as it is the kids. Our kids are not allowed downstairs on Christmas morning until we are ready to go down with them. For years we’ve setup ‘traps’ for them – aluminum foil on the stairs, string attached to bells. This year, I stepped things up with an Arduino powered electronic trip wire.
Parts
This is a pretty simple project, with the major components being readily available cheaply and easily.
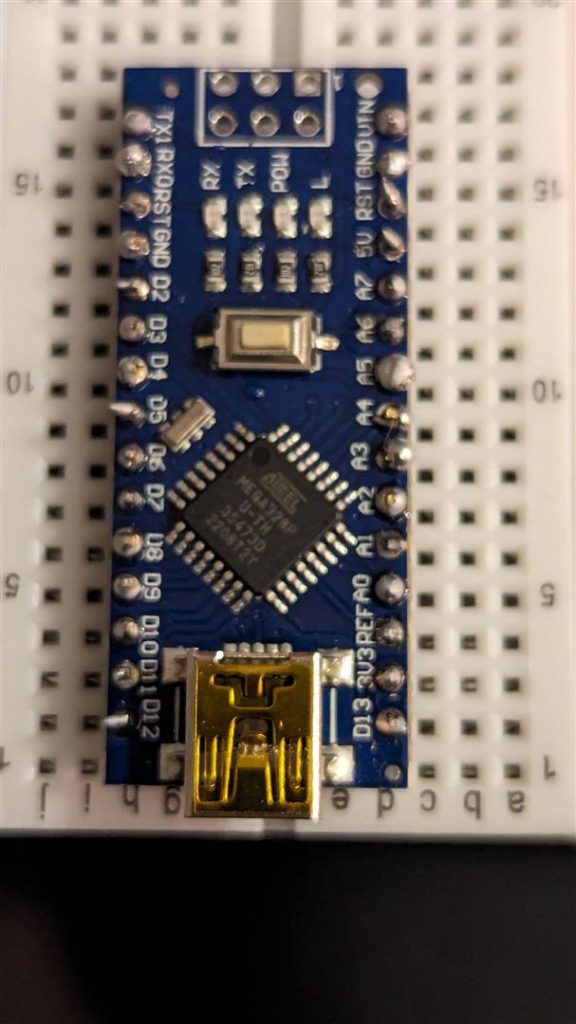
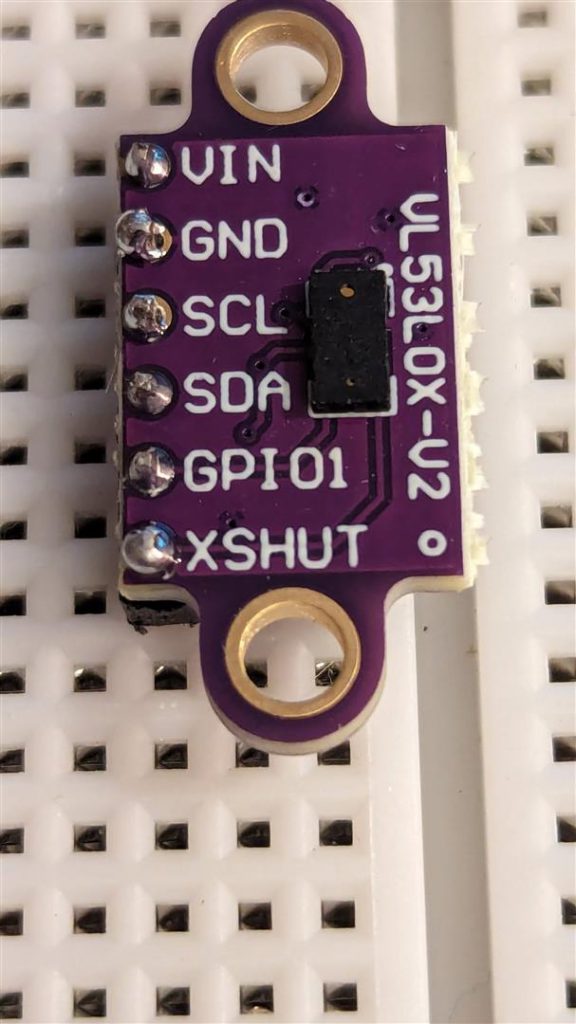
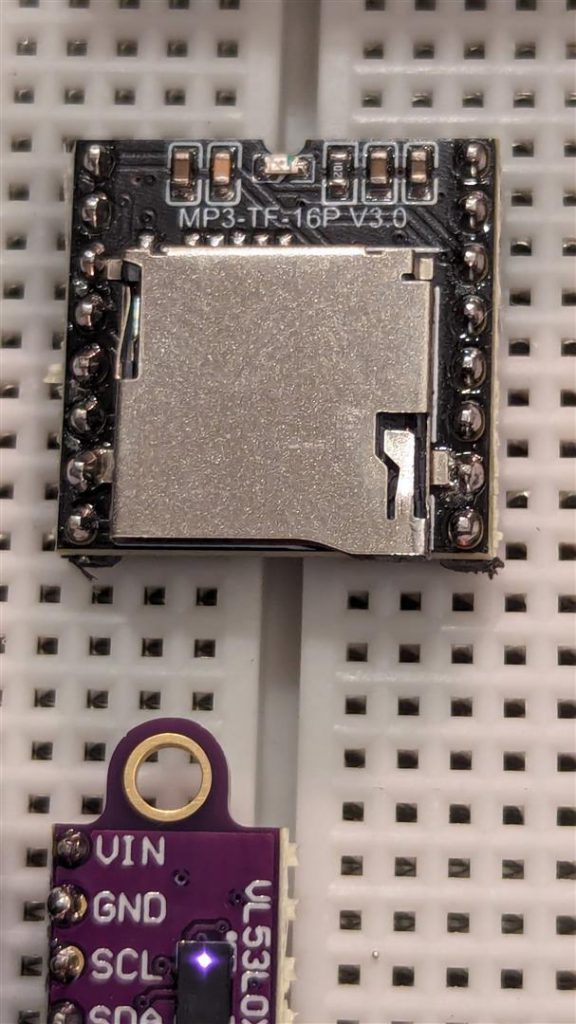
Connecting these together was fairly straight forward. The DF Player has a great library with all the info you need DFRobot / DFRobotDFPlayerMini.
For the distance measureing, the Adafruit library & their examples worked perfectly Adafruit VL53L0X Time of Flight Micro-LIDAR Distance Sensor Breakout.
Breadboarding these out only took a few minutes. For added functionality, I added a simple button. This will be used to set the distance.
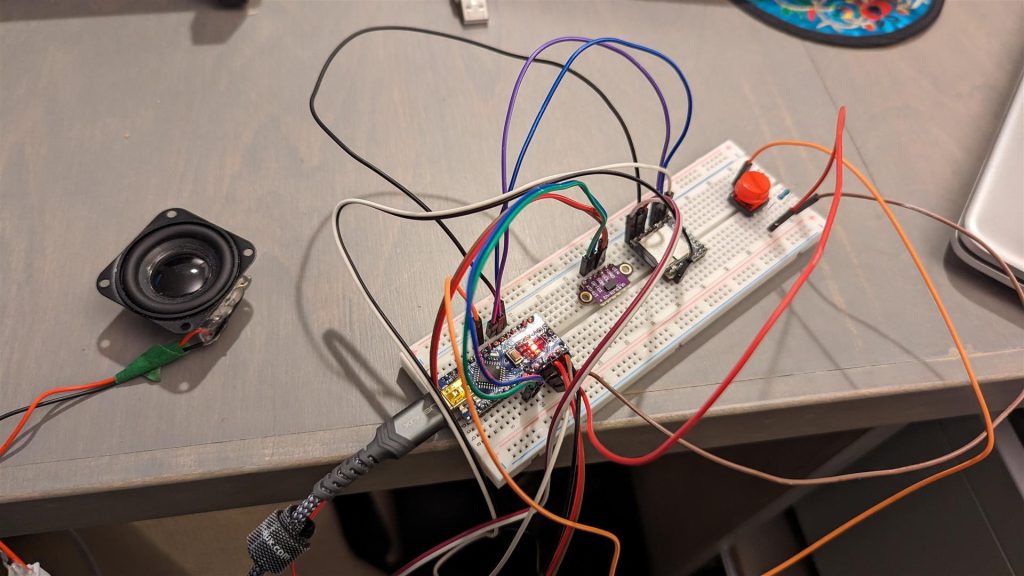
Code
The code got progressively more complicated as I fine-tuned the alerting mechanism. This example uses a fixed alert sound, and some values are hard-coded. Very little is commented.
#include "SoftwareSerial.h"
#include "DFRobotDFPlayerMini.h"
#include "Adafruit_VL53L0X.h"
Adafruit_VL53L0X lox = Adafruit_VL53L0X();
// Use pins 2 and 3 to communicate with DFPlayer Mini
static const uint8_t PIN_MP3_TX = 2; // Connects to module's RX
static const uint8_t PIN_MP3_RX = 3; // Connects to module's TX
SoftwareSerial softwareSerial(PIN_MP3_RX, PIN_MP3_TX);
// Create the Player object
DFRobotDFPlayerMini player;
int baseline_distance = 0;
int last_measurement = 0;
const int button_pin = 5;
int button_state = 0;
bool alert = false;
unsigned long last_alert = 0;
unsigned long current_millis = 0;
int distance_counter = 0;
void setup() {
// Init USB serial port for debugging
Serial.begin(9600);
// Init serial port for DFPlayer Mini
softwareSerial.begin(9600);
Serial.println("Adafruit VL53L0X test.");
if (!lox.begin()) {
Serial.println(F("Failed to boot VL53L0X"));
while(1);
}
// start continuous ranging
lox.startRangeContinuous();
// Start communication with DFPlayer Mini
if (player.begin(softwareSerial)) {
Serial.println("OK");
// Set volume to maximum (0 to 30).
player.volume(20);
// Play the "0001.mp3" in the "mp3" folder on the SD card
player.playMp3Folder(3);
} else {
Serial.println("Connecting to DFPlayer Mini failed!");
}
pinMode(button_pin, INPUT);
}
void loop() {
current_millis = millis();
button_state = digitalRead(button_pin);
if (button_state == HIGH) {
baseline_distance = last_measurement;
}
if (baseline_distance != 0){
/*
Serial.print("baseline_distance in mm: ");
Serial.print(last_measurement);
Serial.print(" / ");
Serial.println(baseline_distance);
*/
if (last_measurement < baseline_distance - 40){
distance_counter = distance_counter + 1;
if (distance_counter > 3 && current_millis > last_alert + 5000){
if (!alert){
alert = true;
last_alert = current_millis;
}
}
}
else {
distance_counter = 0;
}
}
if (alert){
player.playMp3Folder(3);
/*
Serial.print("Alert:");
Serial.print(last_measurement);
Serial.print(" / ");
Serial.println(baseline_distance);
*/
alert = false;
}
if (lox.isRangeComplete()) {
last_measurement = lox.readRange();
}
}
Assembly
Although this took longer than I would like admit, a quick trip to the dollar store for a suitable container was all that was needed to find the right home for this creation.